»–gHÒ§†–« Admin

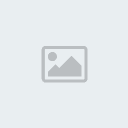
Posts : 92 Join date : 2007-12-19 Age : 32 Location : NY
 | Subject: Drawing player info - Made by Anthrax Tue Dec 25, 2007 3:46 am | |
| Ok this tuturial is going to teach you how to draw your player info in c++ ( using D3D kit ) Creds: me, Kirsch for posting Direct read/write Lets get started First we need to include 2 files, and make a structure holding our players info - Code:
-
#include <stdio.h> #include <print.h>
struct MyInfo {
int team,health,stance;
char *name; };
MyInfo me; ok now we need to create a function to hold all of our shit - Code:
-
void DrawInfo(IDirect3DDevice8 *pD3Ddev) {
} now we need to read all of our information - Code:
-
int base = *(int*)0x96C290;
me.health = *(int*)(base + 226); //reading our health me.name = (char*)(base + 200); //reading our name me.stance = *(int*)(base + 356); //reading our stance me.team = *(int*)(base + 246); //reading our team ok now we need to make some characters so we can draw the info - Code:
-
char mhealth[40]; char mname[40]; char mstance[40]; char mteam[40]; ok now stance and team are read in values, so we need to read those values and tell it what to assign the character to - Code:
-
//reading the stance offsets values and assigning characters text according to the value switch(me.stance) { case 1: case 2: strcpy(mstance,"Stance: Standing"); break; case 13: strcpy(mstance,"Stance: Crouching"); break; case 14: strcpy(mstance,"Stance: Prone"); break; } //reading the team offsets values and assigning characters text according to the value switch(me.team) { case 0: strcpy(mteam,"Team: Green"); break; case 1: strcpy(mteam,"Team: Blue"); break; case 2: strcpy(mteam,"Team: Red"); break; } *note you should log the rest of these values for better results now we need to convert the health integer to a character so we can draw it, and we need to tell it how to draw the name - Code:
-
sprintf(mhealth,"Health: %i",me.health); //converting integers to characters sprintf(mname,"Name: %s",me.name); //telling it how to draw the name info finally, now we draw the text - Code:
-
Print(pD3Ddev, mhealth, "Arial", 16, 0, 5, 5, D3DCOLOR_ARGB( 255, 0, 153, 255)); Print(pD3Ddev, mname, "Arial", 16, 0, 5, 20, D3DCOLOR_ARGB( 255, 0, 153, 255)); Print(pD3Ddev, mstance, "Arial", 16, 0, 5, 35, D3DCOLOR_ARGB( 255, 0, 153, 255)); Print(pD3Ddev, mteam, "Arial", 16, 0, 5, 50, D3DCOLOR_ARGB( 255, 0, 153, 255)); now your finished and your code should look something like this - Code:
-
#include <print.h> #include <stdio.h>
//making a structure to hold our player info struct MyInfo {
int team,health,stance;
char *name; };
MyInfo me;
void DrawInfo(IDirect3DDevice8 *pD3Ddev) { int base = *(int*)0x96C290;
me.health = *(int*)(base + 226); //reading our health me.name = (char*)(base + 200); //reading our name me.stance = *(int*)(base + 356); //reading our stance me.team = *(int*)(base + 246); //reading our team //making char's so we can draw the info as a character char mhealth[256]; char mname[256]; char mstance[256]; char mteam[256]; //reading the stance offsets values and assigning characters text according to the value switch(me.stance) { case 1: case 2: strcpy(mstance,"Stance: Standing"); break; case 13: strcpy(mstance,"Stance: Crouching"); break; case 14: strcpy(mstance,"Stance: Prone"); break; } //reading the team offsets values and assigning characters text according to the value switch(me.team) { case 0: strcpy(mteam,"Team: Green"); break; case 1: strcpy(mteam,"Team: Blue"); break; case 2: strcpy(mteam,"Team: Red"); break; }
sprintf(mhealth,"Health: %i",me.health); //converting integers to characters sprintf(mname,"Name: %s",me.name); //telling it how to draw the name info //drawing all the information Print(pD3Ddev, mhealth, "Arial", 16, 0, 5, 5, D3DCOLOR_ARGB( 255, 0, 153, 255)); Print(pD3Ddev, mname, "Arial", 16, 0, 5, 20, D3DCOLOR_ARGB( 255, 0, 153, 255)); Print(pD3Ddev, mstance, "Arial", 16, 0, 5, 35, D3DCOLOR_ARGB( 255, 0, 153, 255)); Print(pD3Ddev, mteam, "Arial", 16, 0, 5, 50, D3DCOLOR_ARGB( 255, 0, 153, 255));
} | |
|
-TM- V.I.P


Posts : 78 Join date : 2008-01-04
 | Subject: Re: Drawing player info - Made by Anthrax Fri Jan 04, 2008 5:25 am | |
| | |
|
Jtwizzle Admin

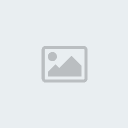
Posts : 163 Join date : 2007-12-21
 | Subject: Re: Drawing player info - Made by Anthrax Mon Jan 07, 2008 12:41 am | |
| Lmao ghost. Nice  | |
|